Create RESTful API using PHP and MySQL
REST stands for Representational State Transfer, it is a software architectural style for handling information over the internet.
API stands for Application Programming Interface, it is a group of functions and processes that allow the creation of applications that access the features or data of an operating system, application, or other services.
REST API is a need because this is the lightest way to perform CRUD (create, read, update, delete) operations by different applications. In this tutorial, we will explain to you how to create RESTful API with CRUD operation using PHP and MySQL.
So let’s implement RESTful API using PHP and MySQL. look folders and files structure:
- create-rest-api-using-php-mysql
- class
- DBConnection.php
- Employee.php.
- emp
- create.php
- read.php
- update.php
- delete.php
- .htaccess
- class
A REST API should use the basic HTTP methods like GET, POST, PUT, DELETE. These method are used according to the requirement:
- GET — retrieve records from database.
- POST — insert new record in the database.
- PUT/PATCH — update existing record in the Database.
- DELETE — delete record from database.
Step 1: Database design and table
Run the following script against MySQL to create the database
employee
table.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
CREATE TABLE `employee` ( `id` int(11) NOT NULL COMMENT 'primary key', `name` varchar(255) NOT NULL COMMENT 'Employee Name', `email` varchar(255) NOT NULL COMMENT 'Email Address', `salary` float(10,2) NOT NULL COMMENT 'employee salary', `age` int(11) NOT NULL COMMENT 'employee age' ) ENGINE=InnoDB DEFAULT CHARSET=latin1 COMMENT='datatable demo table'; ALTER TABLE `employee` ADD PRIMARY KEY (`id`); ALTER TABLE `employee` MODIFY `id` int(11) NOT NULL AUTO_INCREMENT COMMENT 'primary key'; |
Insert sample data into the
employee
table.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
INSERT INTO `employee` (`id`, `name`, `email`, `salary`, `age`) VALUES (1, 'Employee 1 ', 'emp_1@codersmag.com', 3208000.00, 40), (2, 'Employee 2', 'emp_2@codersmag.com', 170750.00, 30), (3, 'Employee 3', 'emp_3@codersmag.com', 86000.00, 25), (4, 'Employee 4', 'emp_4@codersmag.com', 433060.00, 32), (5, 'Employee 5', 'emp_5@codersmag.com', 162700.00, 31), (6, 'Employee 6', 'emp_6@codersmag.com', 372000.00, 25), (7, 'Employee 7', 'emp_7@codersmag.com', 137500.00, 43), (8, 'Employee 8', 'emp_8@codersmag.com', 327900.00, 21), (9, 'Employee 9', 'emp_9@codersmag.com', 205500.00, 35), (10, 'Employee 10', 'emp_10@codersmag.com', 103600.00, 23), (11, 'Employee 11', 'emp_11@codersmag.com', 26584.00, 24), (12, 'Employee 12', 'emp_12@codersmag.com', 26584.00, 25); |
Step 2: Database configuration
Create a class file named
DBConnection.php
inside “class/” folder. You should change your username, password and database name
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 |
<?php /** * @package Database Connection(Config) * * @author CodersMag Team * * @email info@codersmag.com * */ class DBConnection { // HOST NAME private $_dbHostname = "localhost"; // DATABASE NAME private $_dbName = "demo_DB"; // DATABASE USERNAME private $_dbUsername = "root"; // DATABASE PASSWORD private $_dbPassword = ""; // declare variable private $_dbh; // __construct public function __construct() { try { $this->_dbh = new PDO("mysql:host=$this->_dbHostname;dbname=$this->_dbName", $this->_dbUsername, $this->_dbPassword, array(PDO::MYSQL_ATTR_FOUND_ROWS => true)); $this->_dbh->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); } catch(PDOException $e) { echo "Connection failed: " . $e->getMessage(); } } // return PDO: Connection public function returnPDOConnection() { return $this->_dbh; } } ?> |
Step 3: Create class
Create a class file named
Employee.php
inside “class/” folder.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 |
<?php /** * @package Employee class * * @author CodersMag Team * * @email info@codersmag.com * */ include("DBConnection.php"); class Employee { protected $_db; private $_employeeID; private $_name; private $_email; private $_salary; private $_age; public function setEmployeeID($employeeID) { $this->_employeeID = $employeeID; } public function setName($name) { $this->_name = $name; } public function setEmail($email) { $this->_email = $email; } public function setSalary($salary) { $this->_salary = $salary; } public function setAge($age) { $this->_age = $age; } public function __construct() { $this->_db = new DBConnection(); $this->_db = $this->_db->returnPDOConnection(); } // create employee public function createEmp() { try { $sql = 'INSERT INTO employee (name, email, salary, age) VALUES (:name, :email, :salary, :age)'; $data = [ 'name' => $this->_name, 'email' => $this->_email, 'salary' => $this->_salary, 'age' => $this->_age, ]; $stmt = $this->_db->prepare($sql); $stmt->execute($data); $status = $stmt->rowCount(); return $status; } catch (Exception $e) { die("Exception Caught!: ".$e->getMessage()); } } // update employee public function updateEmp() { try { $sql = "UPDATE employee SET name=:name, email=:email, salary=:salary, age=:age WHERE id=:employee_id"; $data = [ 'name' => $this->_name, 'email' => $this->_email, 'salary' => $this->_salary, 'age' => $this->_age, 'employee_id'=> $this->_employeeID ]; $stmt = $this->_db->prepare($sql); $stmt->execute($data); $status = $stmt->rowCount(); return $status; } catch (Exception $e) { die("Exception Caught!: " . $e->getMessage()); } } // getAll employee public function getAllEmp() { try { $sql = "SELECT * FROM employee"; $stmt = $this->_db->prepare($sql); $stmt->execute(); $result = $stmt->fetchAll(\PDO::FETCH_ASSOC); return $result; } catch (Exception $e) { die("Exception Caught!: ".$e->getMessage()); } } // get employee public function getEmp() { try { $sql = "SELECT * FROM employee WHERE id=:employee_id"; $stmt = $this->_db->prepare($sql); $data = [ 'employee_id' => $this->_employeeID ]; $stmt->execute($data); $result = $stmt->fetch(\PDO::FETCH_ASSOC); return $result; } catch (Exception $e) { die("Exception Caught!: ".$e->getMessage()); } } // delete employee public function deleteEmp() { try { $sql = "DELETE FROM employee WHERE id=:employee_id"; $stmt = $this->_db->prepare($sql); $data = [ 'employee_id' => $this->_employeeID ]; $stmt->execute($data); $status = $stmt->rowCount(); return $status; } catch (Exception $e) { die("Exception Caught!: ".$e->getMessage()); } } } ?> |
Step 4: Read Employees
Create a read.php file to read employees list from MySQL database table employee. Call method read() and return all employees list and also single employee if passed employee_id param . We will return employees records as response JSON format.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 |
<?php $reqMethod = $_SERVER["REQUEST_METHOD"]; $json = array(); include('../class/Employee.php'); $emp = new Employee(); switch($reqMethod) { case 'GET': $employeeID = ''; if($_GET['employee_id']) { $employeeID = $_GET['employee_id']; $emp->setEmployeeID($employeeID); $empData = $emp->getEmp(); } else { $empData = $emp->getAllEmp(); } if(!empty($empData)) { $json = json_encode(array('status'=>TRUE, 'empData'=>$empData), true); } else { $json = json_encode(array('status'=>FALSE, 'message'=>'There is no record(s) yet.'), true); } header("Access-Control-Allow-Origin: *"); header("Content-Type: application/json; charset=UTF-8"); header("Access-Control-Allow-Methods: POST"); header("Access-Control-Max-Age: 3600"); header("Access-Control-Allow-Headers: Content-Type, Access-Control-Allow-Headers, Authorization, X-Requested-With"); echo $json; break; default: header("HTTP/1.0 405 Method Not Allowed"); break; } ?> |
Create method read() in class
Employee.php
to fetch employee records from MySQL database table.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 |
// getAll employee public function getAllEmp() { try { $sql = "SELECT * FROM employee"; $stmt = $this->_db->prepare($sql); $stmt->execute(); $result = $stmt->fetchAll(\PDO::FETCH_ASSOC); return $result; } catch (Exception $e) { die("Exception Caught!: ".$e->getMessage()); } } // get employee public function getEmp() { try { $sql = "SELECT * FROM employee WHERE id=:employee_id"; $stmt = $this->_db->prepare($sql); $data = [ 'employee_id' => $this->_employeeID ]; $stmt->execute($data); $result = $stmt->fetch(\PDO::FETCH_ASSOC); return $result; } catch (Exception $e) { die("Exception Caught!: ".$e->getMessage()); } } |
http://localhost/create-rest-api-using-php-mysql/emp/read
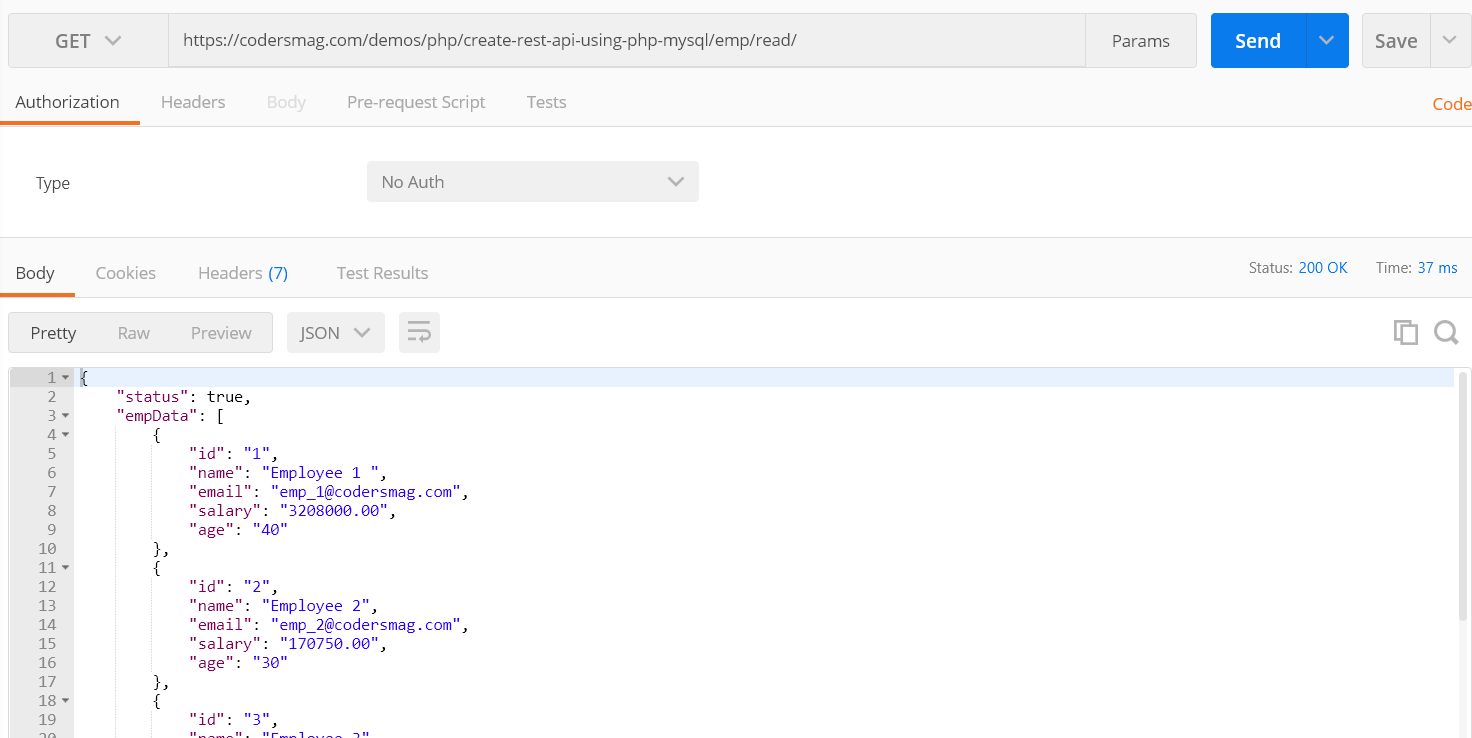
http://localhost/create-rest-api-using-php-mysql/emp/read/1
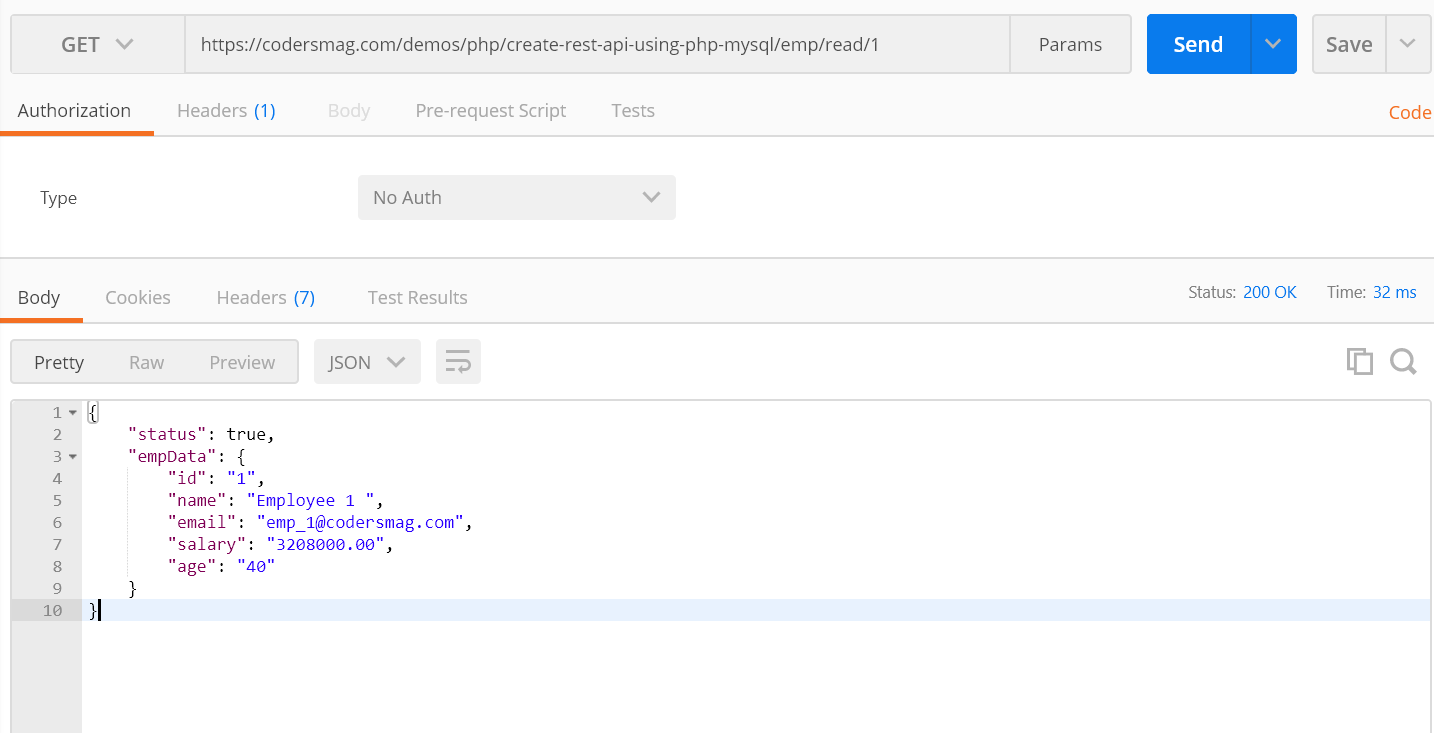
Step 5: Add Employee
Create a create.php file to create employee into MySQL database table employee. Call method create() and handle POST data insert records into MySQL database. We will return response JSON format.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
<?php $reqMethod = $_SERVER["REQUEST_METHOD"]; $json = array(); include('../class/Employee.php'); $emp = new Employee(); switch($reqMethod) { case 'POST': // $name = $_POST['name']; $email = $_POST['email']; $salary = $_POST['salary']; $age = $_POST['age']; // $emp->setName($name); $emp->setEmail($email); $emp->setSalary($salary); $emp->setAge($age); // $empData = $emp->createEmp(); if(!empty($empData)) { $json = json_encode(array('status'=>TRUE, 'msg'=>'Employee created Successfully'), true); } else { $json = json_encode(array('status'=>FALSE, 'msg'=>'Employee creation failed.'), true); } header("Access-Control-Allow-Origin: *"); header("Content-Type: application/json; charset=UTF-8"); header("Access-Control-Allow-Methods: POST"); header("Access-Control-Max-Age: 3600"); header("Access-Control-Allow-Headers: Content-Type, Access-Control-Allow-Headers, Authorization, X-Requested-With"); echo $json; break; default: header("HTTP/1.0 405 Method Not Allowed"); break; } ?> |
Create method create() in class
Employee.php
to save employee record into MySQL database table.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
// create employee public function createEmp() { try { $sql = 'INSERT INTO employee (name, email, salary, age) VALUES (:name, :email, :salary, :age)'; $data = [ 'name' => $this->_name, 'email' => $this->_email, 'salary' => $this->_salary, 'age' => $this->_age, ]; $stmt = $this->_db->prepare($sql); $stmt->execute($data); $status = $stmt->rowCount(); return $status; } catch (Exception $e) { die("Exception Caught!: ".$e->getMessage()); } } |
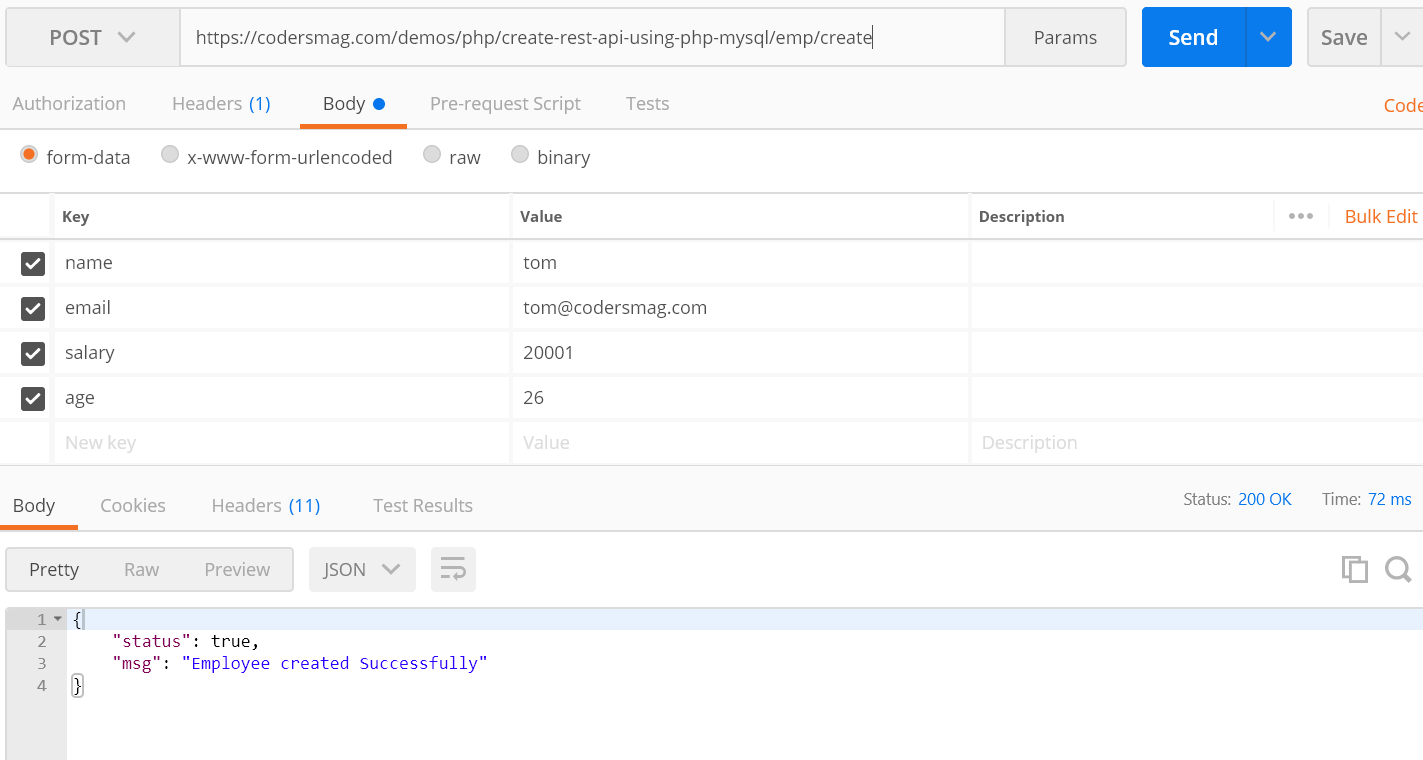
Step 6: Update Employee
Create update.php file to update employee record. We will call method update() from class
Employee.php
and handle employee update functionality through POST method data.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 |
<?php $reqMethod = $_SERVER["REQUEST_METHOD"]; $json = array(); include('../class/Employee.php'); $emp = new Employee(); switch($reqMethod) { case 'POST': $employeeID = $_POST['employee_id']; $name = $_POST['name']; $email = $_POST['email']; $salary = $_POST['salary']; $age = $_POST['age']; $emp->setEmployeeID($employeeID); $emp->setName($name); $emp->setEmail($email); $emp->setSalary($salary); $emp->setAge($age); $empData = $emp->updateEmp(); if(!empty($empData)) { $json = json_encode(array('status'=>TRUE, 'msg'=>'Employee updated Successfully'), true); } else { $json = json_encode(array('status'=>FALSE, 'msg'=>'Employee updation failed.'), true); } header("Access-Control-Allow-Origin: *"); header("Content-Type: application/json; charset=UTF-8"); header("Access-Control-Allow-Methods: POST"); header("Access-Control-Max-Age: 3600"); header("Access-Control-Allow-Headers: Content-Type, Access-Control-Allow-Headers, Authorization, X-Requested-With"); echo $json; default: header("HTTP/1.0 405 Method Not Allowed"); break; } ?> |
Create method update() in class
Employee.php
to update employee record to the MySQL database.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
// update employee public function updateEmp() { try { $sql = "UPDATE employee SET name=:name, email=:email, salary=:salary, age=:age WHERE id=:employee_id"; $data = [ 'name' => $this->_name, 'email' => $this->_email, 'salary' => $this->_salary, 'age' => $this->_age, 'employee_id'=> $this->_employeeID ]; $stmt = $this->_db->prepare($sql); $stmt->execute($data); $status = $stmt->rowCount(); return $status; } catch (Exception $e) { die("Exception Caught!: " . $e->getMessage()); } } |
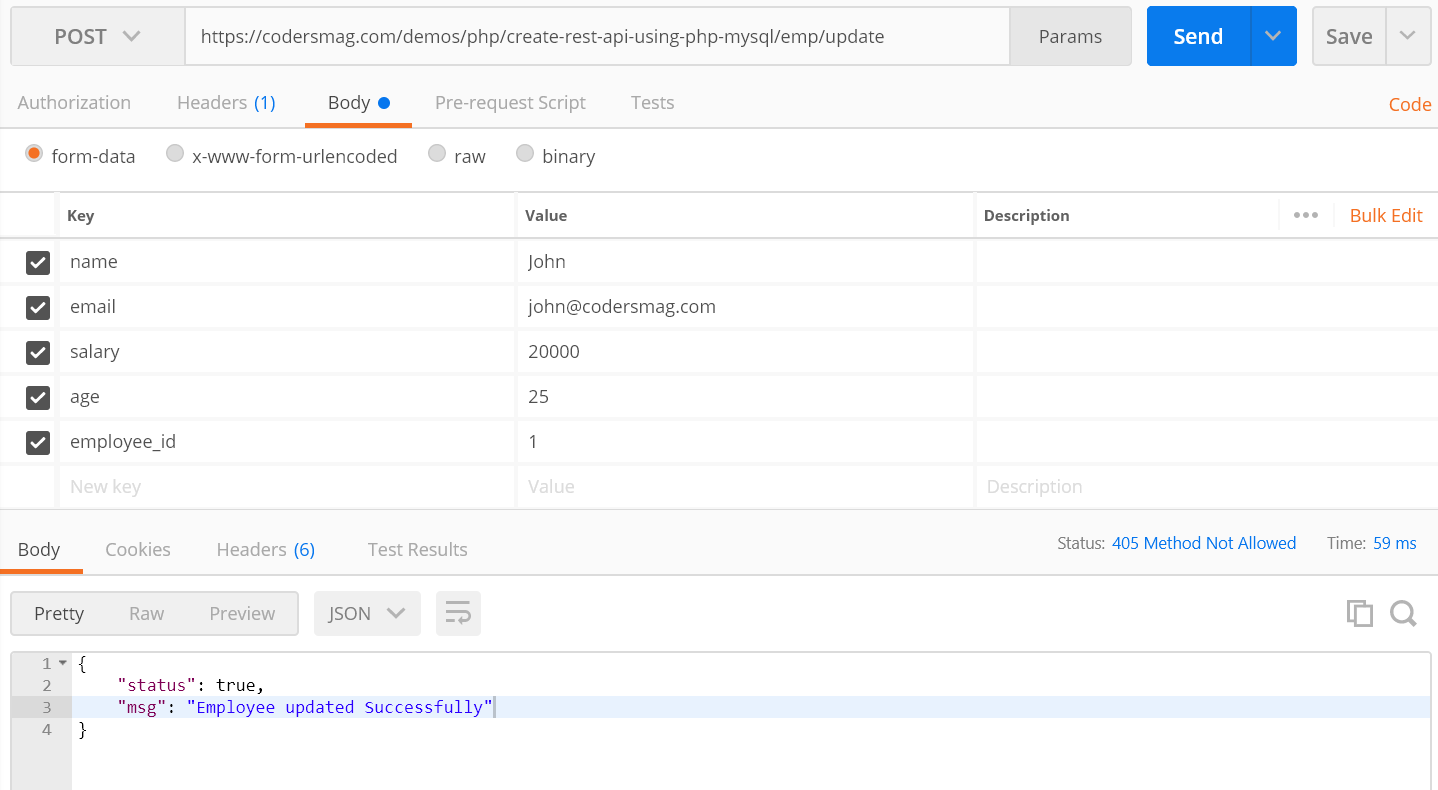
Step 7: Delete Employee
Create a file named delete.php to handle employee delete functionality. We will call method delete() from class
Employee.php
and handle employee delete functionality.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 |
<?php $reqMethod = $_SERVER["REQUEST_METHOD"]; $json = array(); include('../class/Employee.php'); $emp = new Employee(); switch($reqMethod) { case 'GET': $empId = ''; if($_GET['employee_id']) { $employeeID = $_GET['employee_id']; $emp->setEmployeeID($employeeID); } $empData = $emp->deleteEmp(); if(!empty($empData)) { $json = json_encode(array('status'=>TRUE, 'msg'=>'Employee deleted Successfully.'), true); } else { $json = json_encode(array('status'=>FALSE, 'msg'=>'Employee delete failed.'), true); } header("Access-Control-Allow-Origin: *"); header("Content-Type: application/json; charset=UTF-8"); header("Access-Control-Allow-Methods: POST"); header("Access-Control-Max-Age: 3600"); header("Access-Control-Allow-Headers: Content-Type, Access-Control-Allow-Headers, Authorization, X-Requested-With"); echo $json; break; default: header("HTTP/1.0 405 Method Not Allowed"); break; } ?> |
Create method delete() in class
Employee.php
to delete employee record from MySQL database.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
// delete employee public function deleteEmp() { try { $sql = "DELETE FROM employee WHERE id=:employee_id"; $stmt = $this->_db->prepare($sql); $data = [ 'employee_id' => $this->_employeeID ]; $stmt->execute($data); $status = $stmt->rowCount(); return $status; } catch (Exception $e) { die("Exception Caught!: ".$e->getMessage()); } } |
http://localhost/create-rest-api-using-php-mysql/emp/delete/1
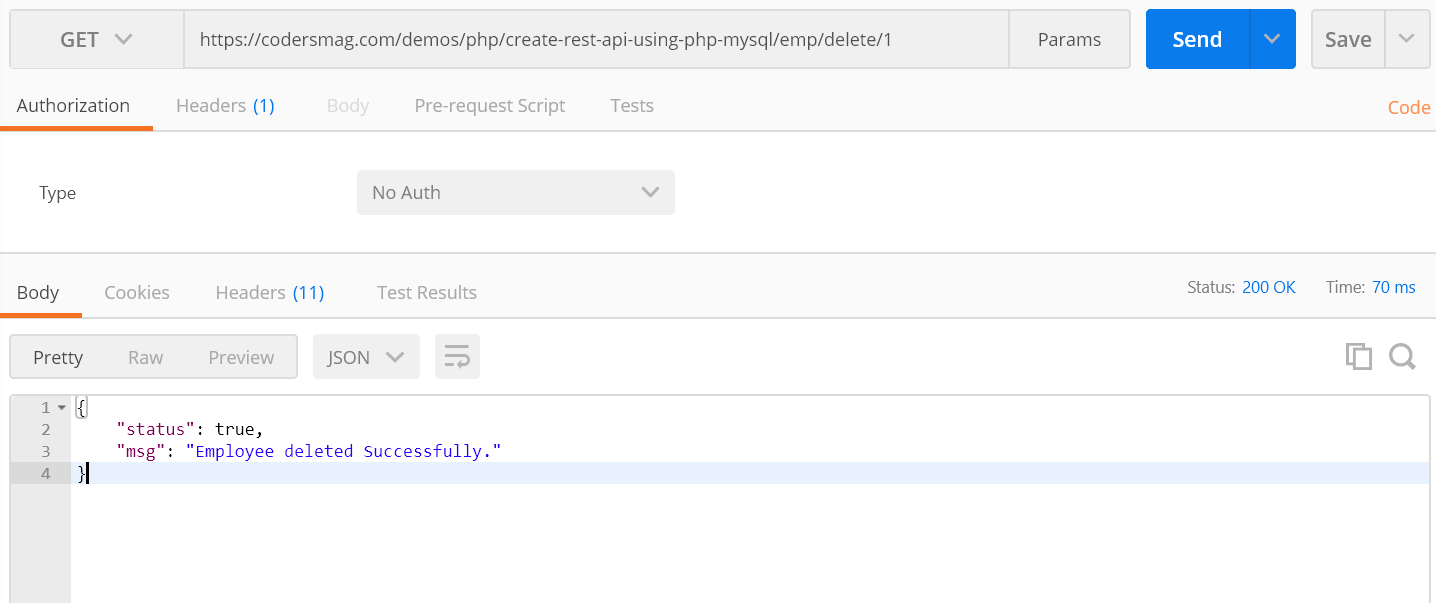