Login with Facebook using PHP SDK
Login with Facebook is a quick and powerful way to integrate registration and login systems on the web application. Facebook is the most popular social network and most of the users have a Facebook account. In this tutorial, you will learn how to build a Login with Facebook using PHP SDK. You can also download the source code of the live example.
So let’s implement Login with Facebook using PHP. look files structure:
- login-facebook-app
- callback
- facebook
- index.php
- facebook
- vendor
- index.php
- callback
Create an app on Facebook
First, you log in Facebook Developer Console. Because when you create an app in the Facebook Developer Console. Then Facebook provides you with some appID and Secret key. With the help of which you can integrate and implement Facebook Login.
Step 1 – Create Facebook App
The following url https://developers.facebook.com/apps and create a new Facebook App.
Step 2 – Create a Facebook app
Create a Facebook app with email and app name:
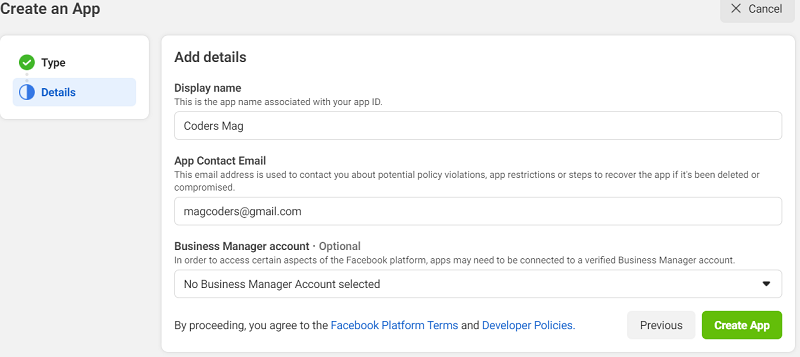
Step 3 – Advanced Setting
Goto
Setting >> Advanced
and add redirect URL: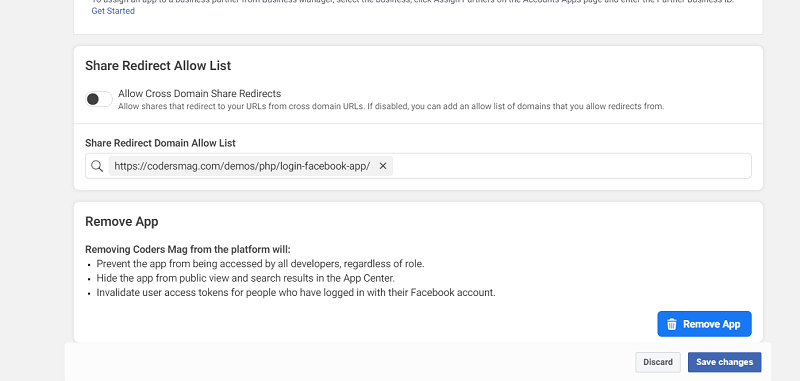
Step 4 – Add valid auth URL
Add valid auth redirect URL. So, click
Facebook Login >> Setting
and add valid auth redirect URL: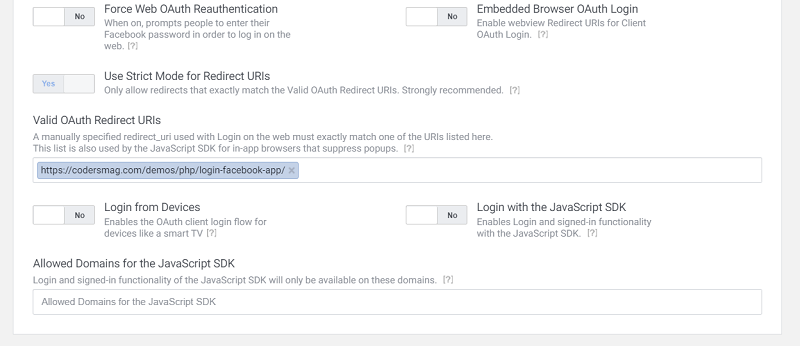
Step 5 – Get App ID and SECRET details:
Go to the Facebook developer dashboard and copy the following App ID and App SECRET:
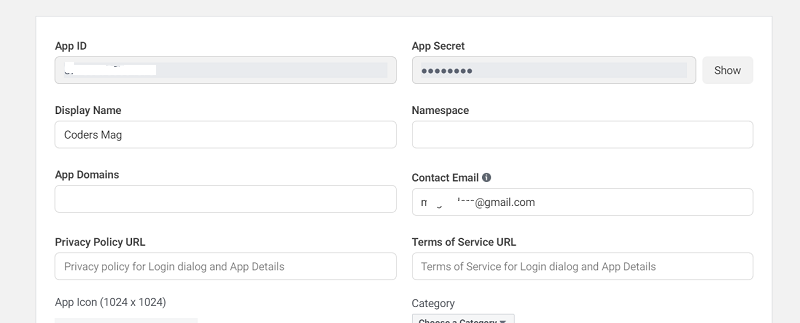
Step 6 – Install Facebook PHP SDK
Install facebook PHP SDK So, Open your command prompt and go to your web application directory and execute the below-given command to install Facebook PHP SDK:
1 2 3 4 5 |
// project directory: login-facebook-app cd login-facebook-app composer require facebook/graph-sdk |
Step 7: Create index.php file
Now on the callback page, we check the user and set session if the user has an account on Facebook.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 |
<?php //facebook sdk require 'vendor/autoload.php'; // session start session_start(); $fb = new Facebook\Facebook([ 'app_id' => 'FB_APPID', 'app_secret' => 'FB_SECRETKEY', 'default_graph_version' => 'v2.10', ]); $helper = $fb->getRedirectLoginHelper(); $permissions = ['email']; // optional try { if (isset($_SESSION['facebook_access_token'])) { $accessToken = $_SESSION['facebook_access_token']; } else { $accessToken = $helper->getAccessToken(); } } catch(Facebook\Exceptions\facebookResponseException $e) { // When Graph returns an error echo 'Graph returned an error: ' . $e->getMessage(); exit; } catch(Facebook\Exceptions\FacebookSDKException $e) { // When validation fails or other local issues echo 'Facebook SDK returned an error: ' . $e->getMessage(); exit; } if (isset($accessToken)) { if (isset($_SESSION['facebook_access_token'])) { $fb->setDefaultAccessToken($_SESSION['facebook_access_token']); } else { // getting short-lived access token $_SESSION['facebook_access_token'] = (string) $accessToken; // OAuth 2.0 client handler $oAuth2Client = $fb->getOAuth2Client(); // Exchanges a short-lived access token for a long-lived one $longLivedAccessToken = $oAuth2Client->getLongLivedAccessToken($_SESSION['facebook_access_token']); $_SESSION['facebook_access_token'] = (string) $longLivedAccessToken; // setting default access token to be used in script $fb->setDefaultAccessToken($_SESSION['facebook_access_token']); } // redirect the user to the account page if it has "code" GET variable if (isset($_GET['code'])) { header('Location: login-facebook-app/callback/facebook/index.php'); } // getting basic info about user try { $account_request = $fb->get('/me?fields=name,first_name,last_name,email'); $requestPicture = $fb->get('/me/picture?redirect=false&height=200'); //getting user picture $picture = $requestPicture->getGraphUser(); $account = $account_request->getGraphUser(); $fbid = $account->getProperty('id'); // To Get Facebook ID $fbfullname = $account->getProperty('name'); // To Get Facebook full name $fbemail = $account->getProperty('email'); // To Get Facebook email $fbpicture = "<img src='".$picture['url']."' class='img-rounded'/>"; # save the user nformation in session variable $_SESSION['fb_id'] = $fbid.'</br>'; $_SESSION['fb_fullname'] = $fbfullname.'</br>'; $_SESSION['fb_email'] = $fbemail.'</br>'; $_SESSION['fb_picture'] = $fbpicture.'</br>'; } catch(Facebook\Exceptions\FacebookResponseException $e) { // When Graph returns an error echo 'Graph returned an error: ' . $e->getMessage(); session_destroy(); // redirecting user back to app login page header("Location: index.php"); exit; } catch(Facebook\Exceptions\FacebookSDKException $e) { // validation fails or other issues echo 'Facebook SDK returned an error: ' . $e->getMessage(); exit; } } else { // replace your web application URL same as added in the developers.Facebook.com/apps e.g. if you used http instead of https and you used $loginURL = $helper->getLoginUrl('https://domainname.com/login-facebook-app/', $permissions); ?> <!DOCTYPE html> <html> <head> <link rel="canonical" href="https://www.codersmag.com/" /> <meta name="author" content="codersmag"> <meta name="description" content="Learn Web Development Tutorials & Web Developer Resources, PHP, MySQL, jQuery, CSS, XHTML, jQuery UI, CSS3, HTML5, HTML, web design, webdesign, with codersmag tutorials. View live demo"> <meta name="keywords" content="codersmag, tutorial codersmag, tutorials, freebies, resources, web development, webdev, demo, PHP, MySQL, jQuery, CSS, XHTML, jQuery UI, CSS3, HTML5, HTML, web design, webdesign, PHP Installation and Configuration, php script, dynamic web content, LAMP, WAMP, XAMPP, installation LAMP server, Installation WAMP server, Installation XAMPP server" /> <meta name="viewport" content="width=device-width, initial-scale=1"> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Download Source Code | Coders Mag</title> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap.min.css"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap-theme.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/js/bootstrap.min.js"></script> <link rel='stylesheet' id='font-awesome-5-css' href='https://codersmag.com/wp-content/themes/v1/css/fontawesome-all.css?ver=5.7.2' type='text/css' media='all' /> <link rel="icon" type="image/ico" href="https://codersmag.com/wp-content/themes/v1/favicon.ico"> <script data-ad-client="ca-pub-1169273815439326" async src="https://pagead2.googlesyndication.com/pagead/js/adsbygoogle.js"></script> </head> <body class=""> <div role="navigation" class="navbar navbar-default navbar-static-top" style="background: #0084B4;"> <div class="container"> <div class="navbar-header"> <button data-target=".navbar-collapse" data-toggle="collapse" class="navbar-toggle" type="button"> <span class="sr-only">Toggle navigation</span> <span class="icon-bar"></span> <span class="icon-bar"></span> <span class="icon-bar"></span> </button> <a href="https://codersmag.com" class="navbar-brand" style="color:#FFF;">CODERSMAG.COM</a> </div> <div class="navbar-collapse collapse"> <ul class="nav navbar-nav"> <li class="active"><a href="https://codersmag.com">Login with Facebook using PHP SDK</a></li> </ul> </div> </div> </div> </body> </html> <?php } ?> |
Step 8: Create index.php file inside folder name “callback/facebook”
Show logged-in user information like Facebook id full name, email, picture, and more
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
<?php session_start(); ?> <!DOCTYPE html> <html> <head> <link rel="canonical" href="https://www.codersmag.com/" /> <meta name="author" content="codersmag"> <meta name="description" content="Learn Web Development Tutorials & Web Developer Resources, PHP, MySQL, jQuery, CSS, XHTML, jQuery UI, CSS3, HTML5, HTML, web design, webdesign, with codersmag tutorials. View live demo"> <meta name="keywords" content="codersmag, tutorial codersmag, tutorials, freebies, resources, web development, webdev, demo, PHP, MySQL, jQuery, CSS, XHTML, jQuery UI, CSS3, HTML5, HTML, web design, webdesign, PHP Installation and Configuration, php script, dynamic web content, LAMP, WAMP, XAMPP, installation LAMP server, Installation WAMP server, Installation XAMPP server" /> <meta name="viewport" content="width=device-width, initial-scale=1"> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Download Source Code | Coders Mag</title> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap.min.css"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap-theme.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/js/bootstrap.min.js"></script> <link rel='stylesheet' id='font-awesome-5-css' href='https://codersmag.com/wp-content/themes/v1/css/fontawesome-all.css?ver=5.7.2' type='text/css' media='all' /> <link rel="icon" type="image/ico" href="https://codersmag.com/wp-content/themes/v1/favicon.ico"> <script data-ad-client="ca-pub-1169273815439326" async src="https://pagead2.googlesyndication.com/pagead/js/adsbygoogle.js"></script> </head> <body class=""> <div role="navigation" class="navbar navbar-default navbar-static-top" style="background: #0084B4;"> <div class="container"> <div class="navbar-header"> <button data-target=".navbar-collapse" data-toggle="collapse" class="navbar-toggle" type="button"> <span class="sr-only">Toggle navigation</span> <span class="icon-bar"></span> <span class="icon-bar"></span> <span class="icon-bar"></span> </button> <a href="https://codersmag.com" class="navbar-brand" style="color:#FFF;">CODERSMAG.COM</a> </div> <div class="navbar-collapse collapse"> <ul class="nav navbar-nav"> <li class="active"><a href="https://codersmag.com">Login with Facebook using PHP SDK</a></li> </ul> </div> </div> </div> </body> </html> |